Kubernetes: How to Deploy Generative AI Models in Minikube

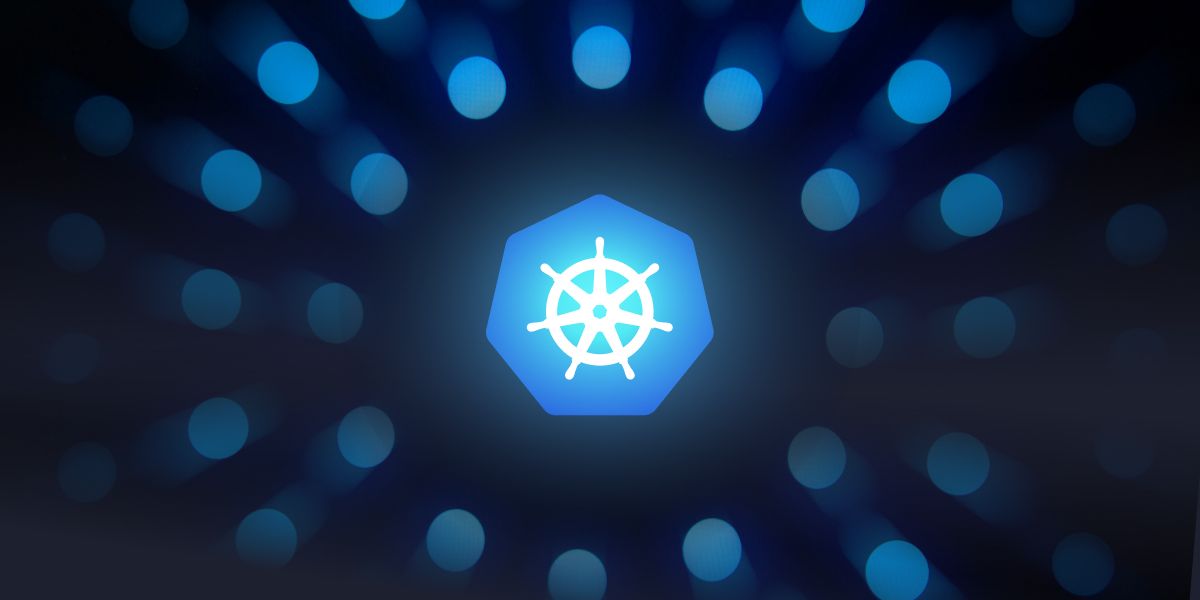

Introduction to Kubernetes and Minikube
Kubernetes has emerged as the go-to platform for container orchestration, allowing developers to efficiently manage, scale, and deploy applications in clusters. Minikube is a lightweight version of Kubernetes that runs locally, perfect for developers to test their apps in a Kubernetes-like environment before pushing them to production.
In this guide, we will walk you through the process of deploying a generative AI model, like GPT-3, in Minikube. By the end, you'll have a working Kubernetes deployment of an AI model, complete with scaling, monitoring, and service exposure.
Why Deploy Generative AI Models in Kubernetes?
Generative AI models are computationally intensive, requiring a robust infrastructure for training and inference. Kubernetes provides a highly scalable and efficient way to manage these resources. Key benefits include:
- Scalability: Kubernetes can automatically scale up/down based on the load.
- Resource Allocation: You can allocate resources like CPUs and GPUs efficiently.
- Automation: Kubernetes automates deployment, management, and scaling tasks.
- Isolation: Kubernetes ensures that each AI model runs in a containerized, isolated environment.
For AI applications that handle large volumes of requests or require continuous availability, Kubernetes is a production-ready solution.
Setting Up Minikube for AI Model Deployment
Before we begin deploying an AI model, we need to set up Minikube on our local machine. Here’s how to do it.
Prerequisites
Ensure you have the following installed on your system:
- Docker
- kubectl
- Minikube
Step-by-Step Guide to Install Minikube
Follow these steps to install and configure Minikube:
- Install Minikube:
# Minikube Installation Instructions
## For Linux (Debian/Ubuntu)
code```
curl -LO https://storage.googleapis.com/minikube/releases/latest/minikube-linux-amd64
sudo install minikube-linux-amd64 /usr/local/bin/minikube
```
# For macOS
code```
brew install minikube
```
# For Windows
code```
choco install minikube
```
- Start Minikube: Once installed, you can start Minikube with a single command:This starts a local Kubernetes cluster running inside a Docker container. Ensure Docker is running on your machine before running this command.
code```minikube start --driver=docker```
- Verify Minikube Installation: Check the Minikube status to ensure the cluster is up and running:Output should show
Running
for all components.
code```minikube status```
- Install kubectl (if not already installed):
code```
# macOS/Linux
brew install kubectl
# Windows
choco install kubernetes-cli
```
- Set kubectl to Use Minikube Context:
code```kubectl config use-context minikube
```
Verify Minikube Setup
To verify everything is working, let's create a simple test deployment:
code```kubectl create deployment hello-minikube --image=k8s.gcr.io/echoserver:1.4```
Expose the deployment via a service:
code```kubectl expose deployment hello-minikube --type=NodePort --port=8080```
Finally, open the Minikube service in your browser:
code```minikube service hello-minikube```
You should see the "EchoServer" running in your browser, which verifies Minikube is working properly.
Understanding Generative AI Models and Their Requirements
Generative AI models, such as GPT-3 or diffusion models, are known for their large size and heavy computational requirements. These models require:
- High computational power (CPUs/GPUs) for inference and training
- Efficient memory management for handling large datasets
- Low-latency networking to handle multiple incoming requests in production environments
Before deploying a generative AI model, it's important to ensure that the Kubernetes cluster can handle these requirements, even in a local setup like Minikube. Minikube can be configured with more resources (CPU, memory) for such tasks.
Configuring Minikube for Resource-Intensive AI Models
If you're planning to run a resource-heavy generative AI model, configure Minikube with more resources:
code```minikube start --cpus 4 --memory 8192 --driver=docker```
This command allocates 4 CPUs and 8GB of RAM to the Minikube cluster, providing more resources for the AI model.
Containerizing Your AI Model for Kubernetes Deployment
Kubernetes requires applications to be packaged as containers. Therefore, the next step is to containerize your generative AI model, such as GPT-3. We’ll use Docker to containerize the model so it can be deployed on Kubernetes.
Here’s how to create a Docker container for your AI model.
Step 1: Create a Simple AI Model API
Let’s create a Python Flask-based API for the generative AI model. This API will expose an endpoint that runs the AI model on the backend.
Create a file app.py
:
code```
from flask import Flask, request, jsonify
import transformers
app = Flask(__name__)
# Load pre-trained GPT-2 model and tokenizer from Hugging Face's transformers library
model_name = "gpt2"
tokenizer = transformers.GPT2Tokenizer.from_pretrained(model_name)
model = transformers.GPT2LMHeadModel.from_pretrained(model_name)
@app.route("/generate", methods=["POST"])
def generate_text():
data = request.json
input_text = data.get("text", "")
# Tokenize input and generate text
inputs = tokenizer.encode(input_text, return_tensors="pt")
outputs = model.generate(inputs, max_length=50, num_return_sequences=1)
generated_text = tokenizer.decode(outputs[0], skip_special_tokens=True)
return jsonify({"generated_text": generated_text})
if __name__ == "__main__":
app.run(host="0.0.0.0", port=5000)
```
Step 2: Create a Dockerfile
The Dockerfile is used to package the application and its dependencies into a container image.
Create a file Dockerfile
:
code```
# Use an official Python runtime as a base image
FROM python:3.8-slim
# Set the working directory
WORKDIR /app
# Copy the current directory contents into the container
COPY . /app
# Install dependencies
RUN pip install flask transformers torch
# Make port 5000 available to the world outside this container
EXPOSE 5000
# Run the application
CMD ["python", "app.py"]
```
Step 3: Build and Test the Docker Image
Now, build the Docker image locally:
code```docker build -t generative-ai-api:latest .```
Run the container locally to ensure it works before deploying it to Minikube:
code```docker run -p 5000:5000 generative-ai-api:latest```
Test the API using curl
or Postman:
code```curl -X POST http://localhost:5000/generate -H "Content-Type: application/json" -d '{"text": "Once upon a time"}'```
If the API returns a generated text response, the container is working correctly.
Pushing Docker Images to a Container Registry
Once you've confirmed that the container works locally, the next step is to push the Docker image to a container registry, so it can be pulled by the Minikube cluster.
Step 1: Tag the Docker Image
Tag the image to match the naming convention of your Docker Hub repository:
code```docker tag generative-ai-api:latest <your-dockerhub-username>/generative-ai-api:latest```
Step 2: Log In to Docker Hub
Log in to your Docker Hub account using the following command:
code```docker login```
Step 3: Push the Image to Docker Hub
Push the image to Docker Hub so it can be accessed from any Kubernetes cluster:
code```docker push <your-dockerhub-username>/generative-ai-api:latest```
The image will now be available in your Docker Hub account, ready to be deployed in Minikube.
Deploying Your AI Model in Minikube
Now that we have our generative AI model containerized and pushed to a container registry, the next step is to deploy it in Minikube using Kubernetes configurations. We will use Kubernetes Deployments to manage our AI model, and expose the model using Kubernetes Services.
Step 1: Create a Kubernetes Deployment
A Kubernetes deployment manages the running instances of your AI model container. It ensures that the correct number of instances are always up and running, and can automatically restart them in case of failure.
Create a new YAML file called deployment.yaml
for the deployment configuration:
code```
apiVersion: apps/v1
kind: Deployment
metadata:
name: generative-ai-deployment
spec:
replicas: 2 # Number of instances
selector:
matchLabels:
app: generative-ai
template:
metadata:
labels:
app: generative-ai
spec:
containers:
- name: generative-ai-container
image: <your-dockerhub-username>/generative-ai-api:latest # Use your Docker image
ports:
- containerPort: 5000
resources:
limits:
memory: "512Mi"
cpu: "0.5"
requests:
memory: "256Mi"
cpu: "0.25"
```
Step 2: Apply the Deployment
Now that you have the deployment configuration ready, apply it to the Minikube cluster using kubectl
:
code```kubectl apply -f deployment.yaml```
This command deploys two replicas of the AI model container on Minikube.
Step 3: Verify the Deployment
To check if the deployment is running correctly, you can use the following command:
code```kubectl get deployments```
This will show the status of your deployment and how many replicas are running. If everything is set up properly, you should see two pods up and running:
code```kubectl get pods```
This command will list the pods, and you should see two pods with names starting with generative-ai-deployment
that are in a Running
state.
Exposing the AI Model as a Kubernetes Service
Now that your AI model is running in Kubernetes, it’s time to expose it so that external applications can access it. We’ll expose the deployment as a Kubernetes Service.
Step 1: Create a Service YAML Configuration
Services in Kubernetes expose your application to external traffic or other internal applications within the cluster. Here, we’ll create a service to expose the AI model API.
Create a new file called service.yaml
:
code```
apiVersion: v1
kind: Service
metadata:
name: generative-ai-service
spec:
type: NodePort # Allows access via a port on the node
selector:
app: generative-ai
ports:
- protocol: TCP
port: 5000 # The port that the service will expose
targetPort: 5000 # The port inside the container
nodePort: 30007 # NodePort for external access (Minikube range: 30000-32767)
```
Step 2: Apply the Service Configuration
Once the service configuration is created, apply it to Minikube using kubectl
:
code```kubectl apply -f service.yaml```
This will create a service that routes traffic from NodePort
30007 to the AI model containers running in your deployment.
Step 3: Access the Service in Minikube
To access the running AI model through the service, use the following command:
code```minikube service generative-ai-service```
This command will open the service in your default web browser, where you can access the AI model’s API. You can also use curl
to test the endpoint:
code```curl -X POST http://$(minikube ip):30007/generate -H "Content-Type: application/json" -d '{"text": "Once upon a time"}'```
This will return a generated text response from the AI model.
Scaling Your AI Model in Minikube
One of the key benefits of Kubernetes is its ability to scale applications seamlessly. You can easily increase or decrease the number of replicas of your AI model based on demand.
Step 1: Manually Scale the Deployment
To scale your AI model up or down, use the kubectl scale
command. For example, to scale the deployment to 5 replicas:
code```kubectl scale deployment generative-ai-deployment --replicas=5```
This command increases the number of pods running the AI model to 5. You can verify this by running:
code```kubectl get pods```
You should see five pods in the Running
state.
Step 2: Configure Auto-Scaling
Kubernetes also allows for auto-scaling based on CPU or memory usage. You can configure the Horizontal Pod Autoscaler (HPA) to automatically increase or decrease the number of pods based on usage.
To create an HPA for the AI model, use the following command:
code```kubectl autoscale deployment generative-ai-deployment --cpu-percent=50 --min=2 --max=10```
This creates an HPA that scales the AI model between 2 and 10 replicas, depending on CPU usage. If the CPU usage exceeds 50%, Kubernetes will add more replicas to handle the load.
Step 3: Monitoring the Autoscaler
To check the status of the autoscaler and the scaling decisions it makes, use:
code```kubectl get hpa```
This will show the current CPU utilization and the number of replicas managed by the autoscaler.
Conclusion: Bringing AI Models to Production with Kubernetes
In this tutorial, we walked through how to deploy a generative AI model in Minikube using Kubernetes, containerizing the model, exposing it via a service, and scaling it up based on demand. This workflow mirrors a production-grade setup, giving you a solid foundation for deploying AI models in real-world environments.
Kubernetes is ideal for AI workloads because of its scalability, resource management, and resilience. By using Minikube for local development, you can ensure your AI models are production-ready before deploying them to full-scale Kubernetes clusters.